⭐ Bare Minimum Requirements
⬛ 서버 코드 작성
읽기 routes/article/read.js
const { readAll, readOne } = require('../../model')
module.exports = async function (app, opts) {
app.get('/', async function (request, reply) {
// 게시글 전체 조회
const result = await readAll()
// 성공 결과 코드 및 게시글 데이터 반환
reply
.code(200)
.header('Content-type', 'application/json')
.send(result)
})
app.get('/:id', async function (request, reply) {
const id = request.params.id
// 특정 ID의 게시글 조회
const result = await readOne(id)
// 해당 ID를 가진 게시글이 있을 때
if(result) {
// 성공 결과 코드 및 게시글 데이터 반환
reply
.code(200)
.header('Content-type', 'application/json')
.send(result)
}
// 해당 ID를 가진 게시글이 없을 때
else {
// 404 실패 코드 및 "Article Not Found" 메시지 반환
reply
.code(404)
.header('Content-type', 'application/json')
.send("Article Not Found")
}
})
}
쓰기 routes/article/create.js
const { createOne, isValid } = require('../../model')
module.exports = async function (app, opts) {
app.post('/', async function (request, reply) {
const body = request.body
// requestBody validation 실패시
if(!isValid(body)) {
// 400 실패 코드 및 "Bad Request" 메시지 반환
reply
.code(400)
.header('Content-type', 'application/json')
.send("Bad Request")
return;
}
// requestBody 데이터로 신규 게시글 생성
const result = await createOne(body)
// 게시글이 생성되었을 때
if(result) {
// 생성 결과 코드 및 게시글 데이터 반환
reply
.code(201)
.header('Content-type', 'application/json')
.send(result)
// 게시글이 생성되지 않았을 때
} else {
reply
// 500 실패 코드 및 "Server Error" 메시지 반환
.code(500)
.header('Content-type', 'application/json')
.send("Server Error")
}
})
}
삭제 routes/article/delete.js
const { deleteOne } = require('../../model')
module.exports = async function (app, opts) {
app.delete('/:id', async function (request, reply) {
// 특정 ID의 게시글 삭제
const result = await deleteOne(request.params.id)
// 해당 ID를 가진 게시글을 삭제했을 때
if(result) {
// 삭제 결과 코드 및 "Delete Success" 메시지 반환
reply
.code(204)
.header('Content-type', 'application/json')
.send("Delete Success")
}
// 해당 ID를 가진 게시글이 없을 때
else {
// 404 실패 코드 및 "Article Not Found" 메시지 반환
reply
.code(404)
.header('Content-type', 'application/json')
.send("Article Not Found")
}
})
}
수정 routes/article/update.js
const { updateOne, isValid } = require('../../model')
module.exports = async function (app, opts) {
app.put('/:id', async function (request, reply) {
// requestBody validation 실패시
if(!isValid(request.body)) {
// 400 실패 코드 및 "Bad Request" 메시지 반환
reply
.code(400)
.header('Content-type', 'application/json')
.send("Bad Request")
return;
}
// 특정 ID의 게시글을 requestBody 데이터로 수정
const result = await updateOne(request.params.id, request.body)
// 해당 ID를 가진 게시글을 수정했을 때
if(result) {
// 성공 결과 코드 및 게시글 데이터 반환
reply
.code(200)
.header('Content-type', 'application/json')
.send(result)
}
else {
// 404 실패 코드 및 "Article Not Found" 메시지 반환
reply
.code(404)
.header('Content-type', 'application/json')
.send("Article Not Found")
}
})
}
⬛ 결과 확인하기
게시글 목록 조회
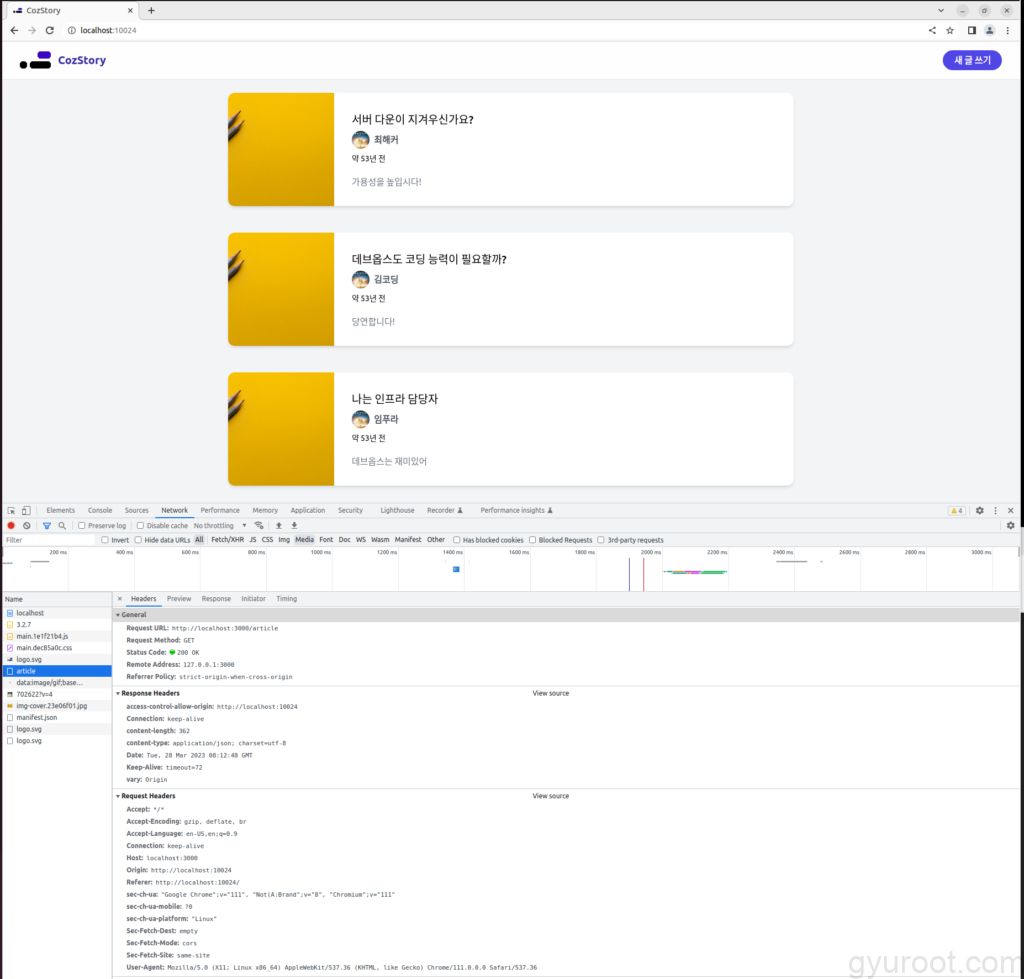
특정 게시글 조회
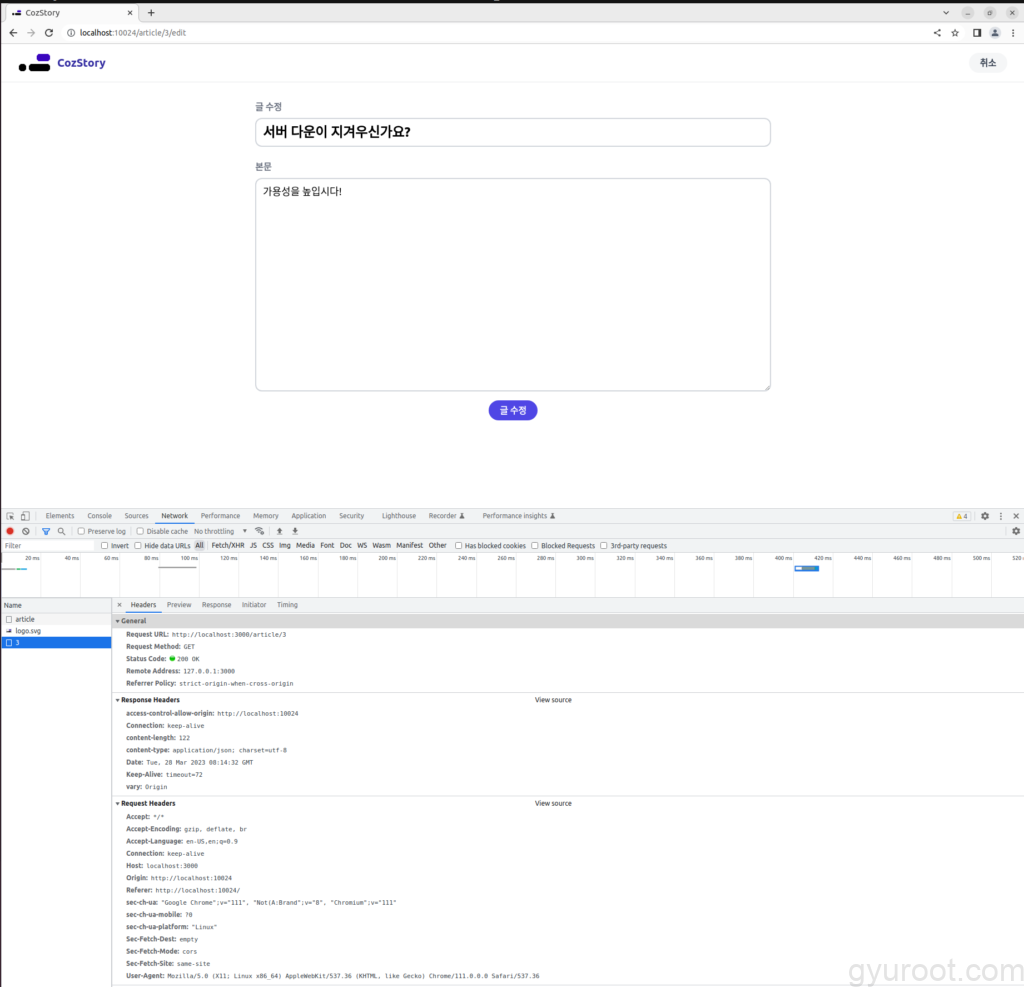
게시글 생성
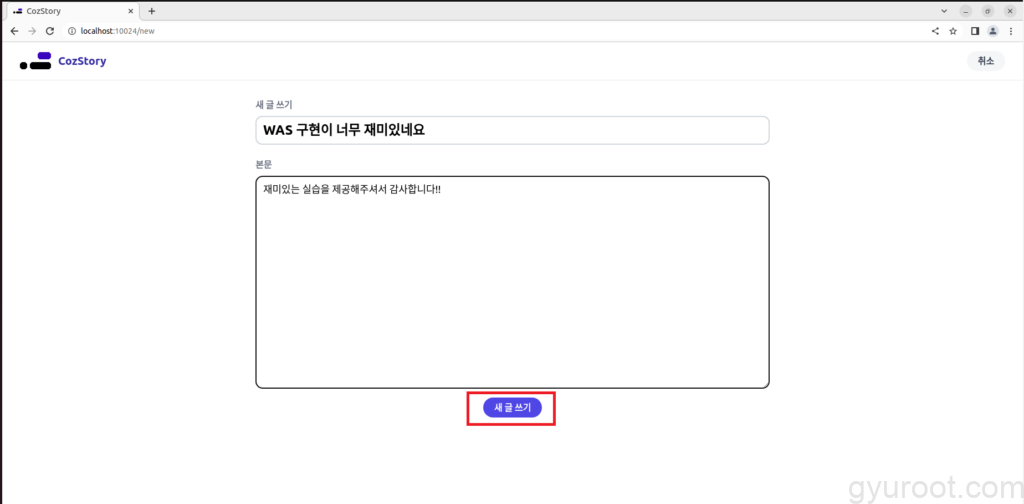
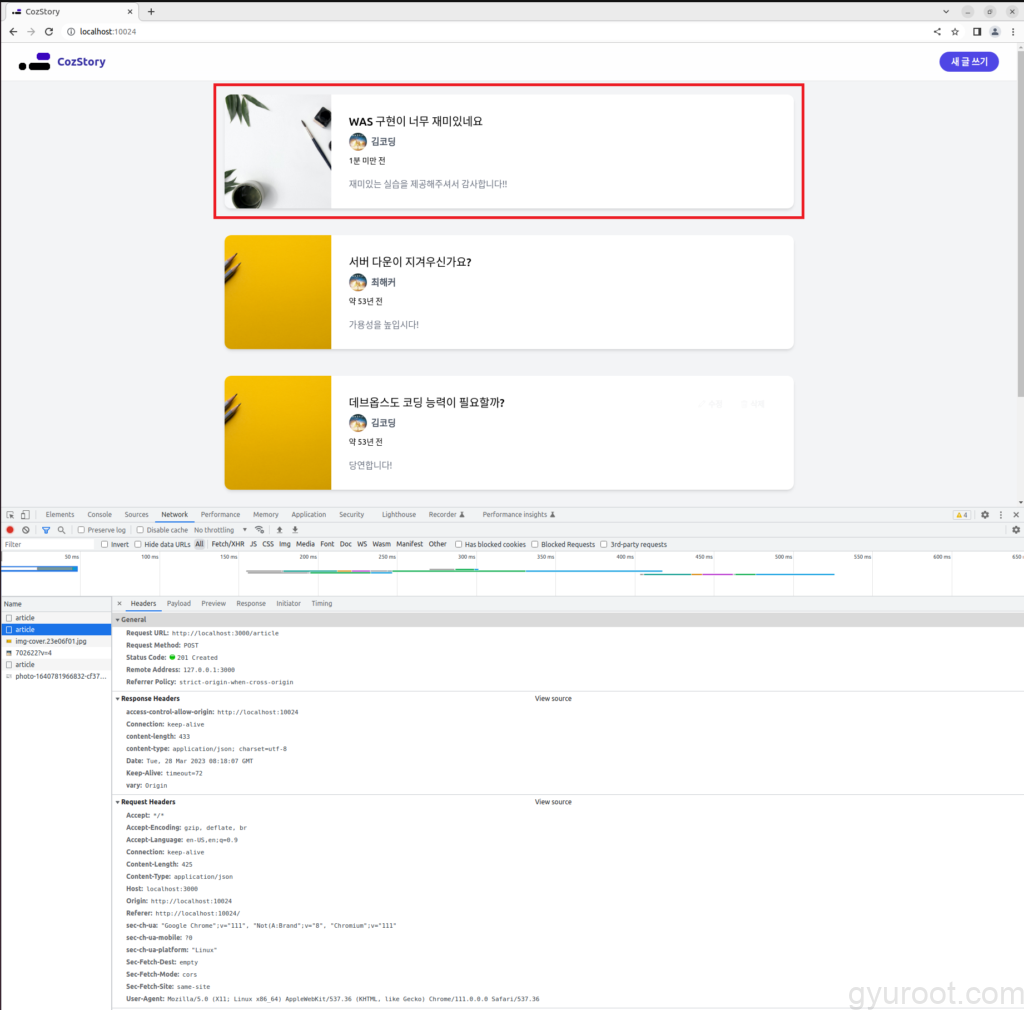
게시글 수정
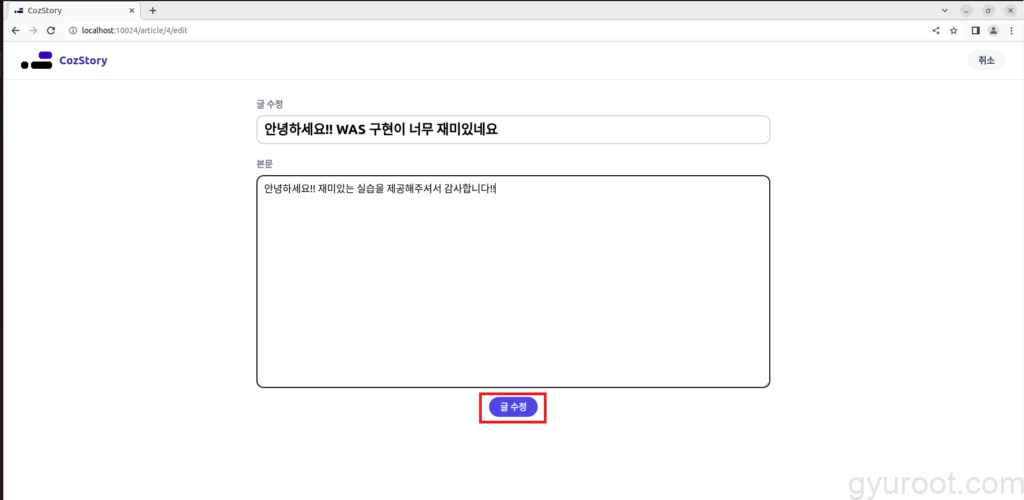
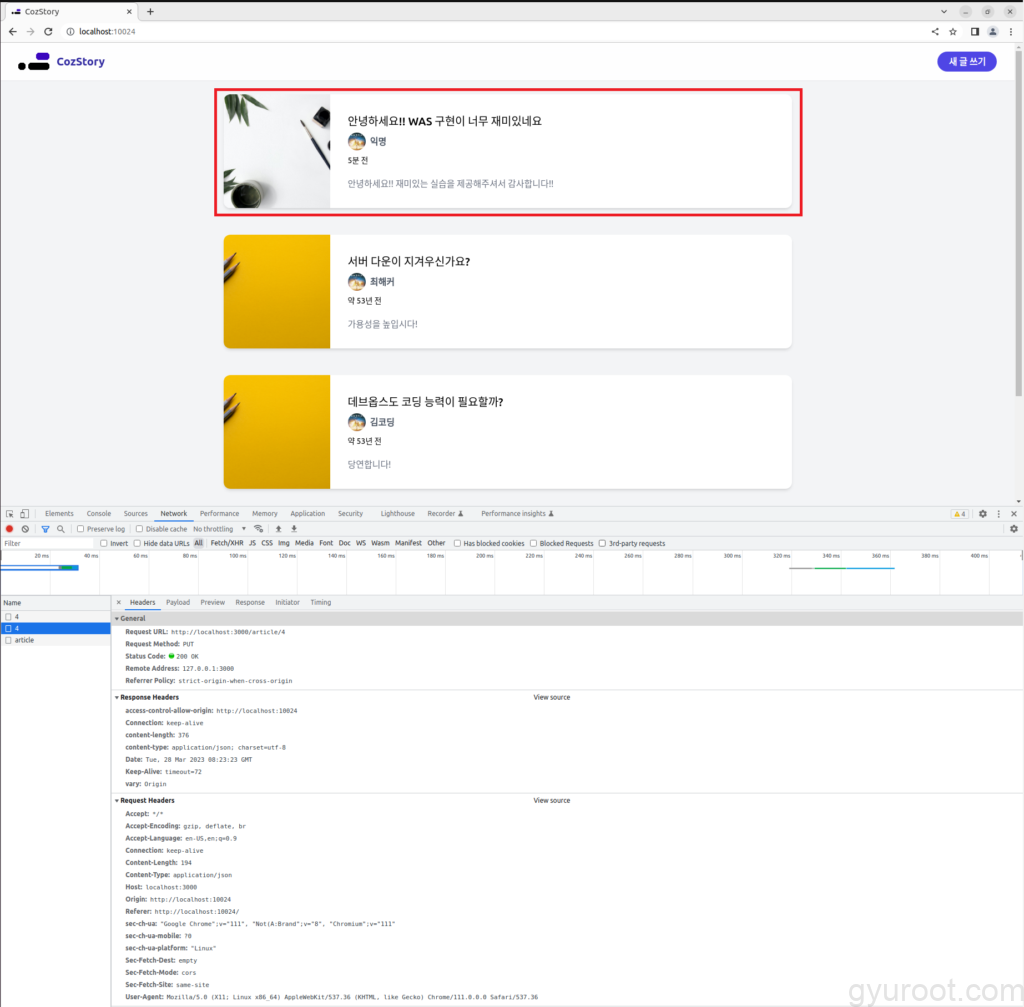
게시글 삭제
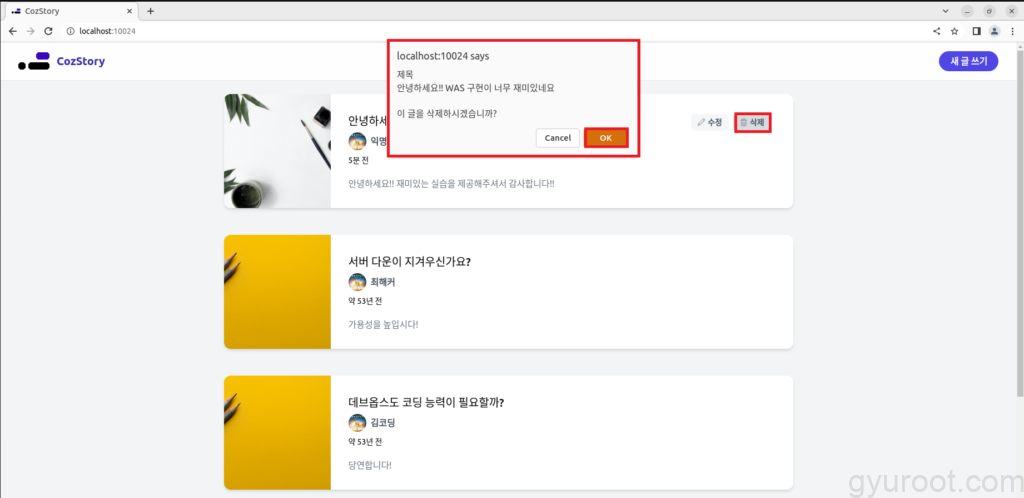
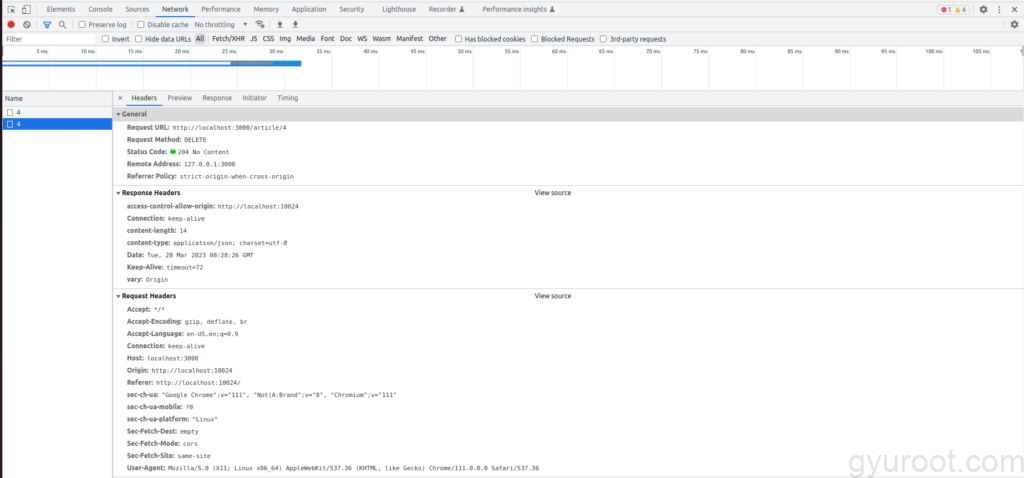